While working on TFS with Dynamics AX 2012, sometimes I get the following error when I try to check-out a certain file.
This error occured because either you had synchronized your workspace with the files that were not in consistent state or you had checked-in your files which had some errors in .xpo code, like a node was missing etc.
The complete error message is as follows:
The file D:\TFS-EDM\usr\Data Sets\EdmPreviousInstitutionsGridDS.xpo was not correctly imported to the model store in a previous synchronization. Any updates of the file is blocked from AX. Open Version Control, Synchronization log to re-import the file.
To fix this error, open visual studio, go to Team Explorer (Menus-> View-> Team Explorer), and connect to your project with the TFS. After connecting to the project, just locate the file that you want to check out. Right click the file, and click "Check out for Edit".
Now go back to AOT, right click on that file, and click "Undo Check-out". Now you can check this file out successfully by clicking on the file and clicking "Check-out".
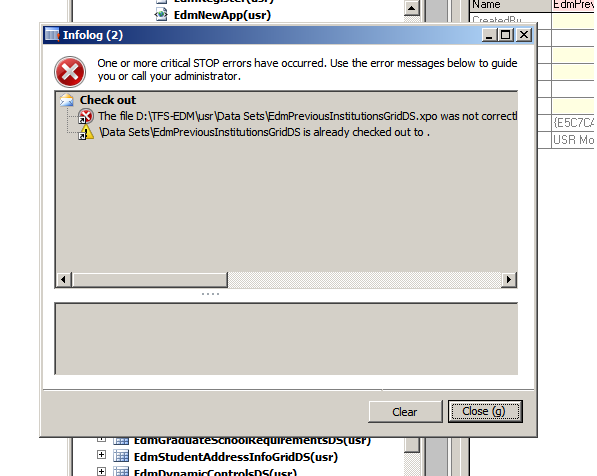
This error occured because either you had synchronized your workspace with the files that were not in consistent state or you had checked-in your files which had some errors in .xpo code, like a node was missing etc.
The complete error message is as follows:
The file D:\TFS-EDM\usr\Data Sets\EdmPreviousInstitutionsGridDS.xpo was not correctly imported to the model store in a previous synchronization. Any updates of the file is blocked from AX. Open Version Control, Synchronization log to re-import the file.
To fix this error, open visual studio, go to Team Explorer (Menus-> View-> Team Explorer), and connect to your project with the TFS. After connecting to the project, just locate the file that you want to check out. Right click the file, and click "Check out for Edit".
Now go back to AOT, right click on that file, and click "Undo Check-out". Now you can check this file out successfully by clicking on the file and clicking "Check-out".